“A data structure is a storage technique that is used to store and organize data. It is a way of arranging data on a computer memory so that it can be accessed and updated efficiently.”
Data Structures are typically used to organize, process, retrieve and store data on computers memory for efficient use. Having the right understanding and using the right data structures helps software engineers write the right code.
There are two types of Data Structures
Linear Data structure: If the elements of a data structure result in a sequence or a linear order then it is called a linear data structure. Every data element is connected to its next element and sometimes also to its previous element in a sequential manner. Example - Arrays, Linked Lists, Stacks, Queues, etc.
Non-linear Data structure: If the elements of a Data structure result in a way that the traversal of nodes is not done in a sequential manner, then it is a Non-linear data structure. Its elements are not sequentially connected, and every element can attach to another element in multiple ways. Example - Hierarchical data structure like trees.
Important of data structures
- Data structures are a key component of Computer Science and help in understanding the nature of a given problem at a deeper level. They’re widely utilized in Artificial Intelligence, operating systems, graphics, and other fields. If the programmer is unfamiliar with data structure and Algorithms, they may be unable to write efficient data-handling code.
- A strong grasp of this is of significance if you want to learn how to organize and assemble data and solve real-life problems
- Good understanding of data structures will help you write efficient code in your day to day work
As we have understood the importance of data structures, we should also remember that knowing when to use the right one is also equally important. Not using the right data structure will create a lot of problems with efficiency and performance.
Classification of Data Structure
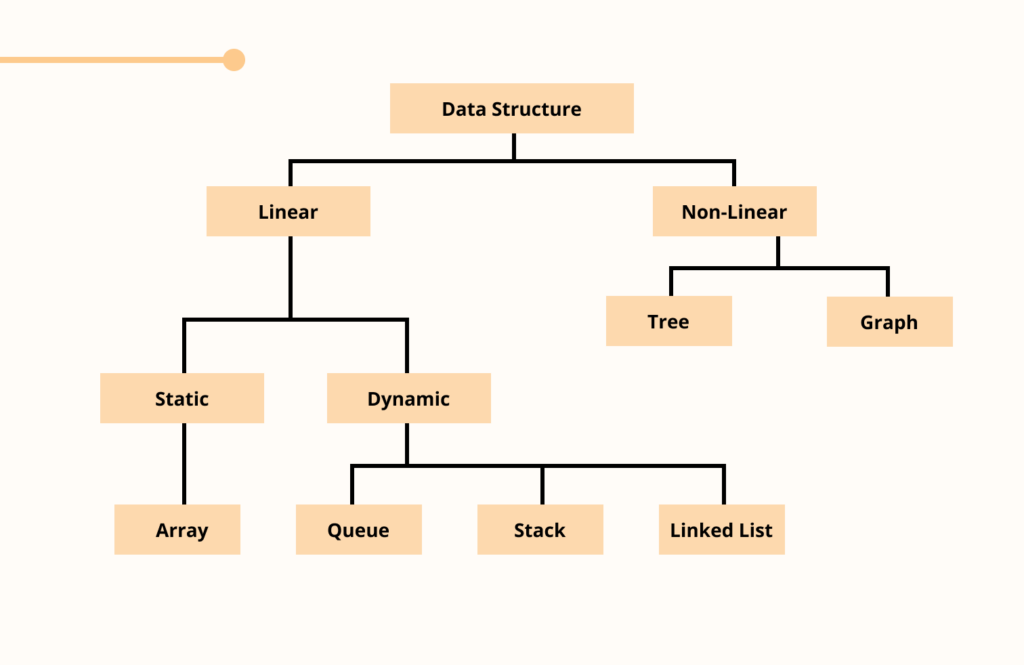
Short Summary
Array: An array is a collection of similar data elements stored at contiguous memory locations. It is the simplest data structure where each data element can be accessed directly by only using its index number.
Queue: A collection of items in which only the earliest added item may be accessed. Basic operations are add (to the tail) or enqueue and delete (from the head) or dequeue. Delete returns the item removed. Also known as "first-in, first-out" or FIFO.
Stack: linear data structure that follows a particular order in which the operations are performed. The order may be LIFO(Last In First Out) or FILO(First In Last Out). LIFO implies that the element that is inserted last, comes out first and FILO implies that the element that is inserted first, comes out last.
Tree: A tree data structure is a hierarchical structure that is used to represent and organize data in a way that is easy to navigate and search. It is a collection of nodes that are connected by edges and has a hierarchical relationship between the nodes.
Graph: A Graph is a non-linear data structure consisting of vertices and edges. The vertices are sometimes also referred to as nodes and the edges are lines or arcs that connect any two nodes in the graph. More formally a Graph is composed of a set of vertices( V ) and a set of edges( E ). The graph is denoted by G(E, V).
In our next article, we will explore “Stack” data structure in detail. Till that time, Namaste! 🙏.